Common String Methods
Remember from our discussion above, a String is considered an "object" in JavaScript, and thus, has built-in "methods" (just another word for "functions"). I do not intend to cover all of the String methods (you can find them here) nor do I intend to explain any one of these in extreme detail. My intention is to show you some of the common methods and the basics of how they work so when it comes time to use them, you will already have some familiarity.
Here are the string methods that I find myself using most.
- replaceAll()
- toUpperCase()
- substring()
- trim()
- match()
replaceAll
This method comes in handy when you want to replace multiple occurrences of a value in a string.
const myString = "My Dog jumped on the bed. My dog is a bad Dog.";
// Remember, a string primitive like `myString` is immutable, so we are
// not editing it directly. We are assigning the result to a new variable
const newString = myString.replaceAll("Dog", "Cat");
console.log(newString); // My Cat jumped on the bed. My dog is a bad Cat.
Notice anything wrong here? We only replaced the uppercase version of Dog
! This is where regular expressions come in handy. The replaceAll()
method accepts either a string OR a regular expression for its first argument.
const myString = "My Dog jumped on the bed. My dog is a bad Dog.";
const newString = myString.replaceAll(/[Dd]{1}og/g, "cat");
console.log(newString); // My cat jumped on the bed. My cat is a bad cat.
We wrote /[Dd]{1}og/g
as our regular expression which will match exactly 1 character that is either D
or d
followed by og
. The g
character at the end is not something we talked about earlier, but it represents the "global" flag (i.e. match ALL occurrences of this pattern rather than just the first). For more on regular expression flags, you can read this.
As you can see above, we replaced all occurrences of "dog" (uppercase and lowercase) by using a single expression.
toUpperCase
This method does exactly what its name suggests. It capitalizes every letter in a given string.
const myString = "some string";
console.log(myString.toUpperCase()); // SOME STRING
You'll see how this method can be useful in the next method, substring()
.
substring
If you look in the documentation, you'll see the following two methods.
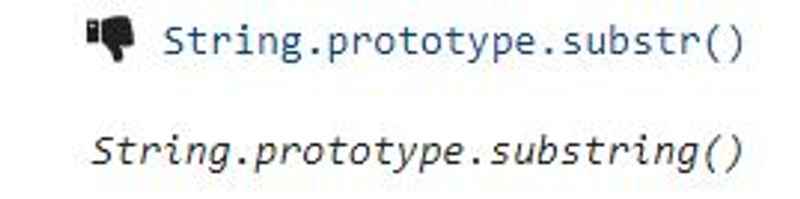
Notice that thumbs down icon? That means that the method is "deprecated" (no longer supported). Deprecated methods will still work in most cases, but may become unsupported by certain browsers over time.
While I did accidentally use substr()
in a prior lesson during one of our code challenges, you should always use substring()
because it is not deprecated.
This method is great when you need to isolate a section of a string.
// Index 0123456789 ......
const myString = "the programmer had a lot of bugs in his house";
const substr = myString.substring(4, 14);
console.log(substr); // programmer
Notice the index values that I placed above the first couple letters in myString
. The substring()
method takes two arguments–the starting index and ending index. In this example, we start at index 4
and end at index 14
. If you would have skipped the first argument (i.e. myString.substring(4)
), the method would return the entire string starting at index 4
.
This method can come in handy in lots of situations, but here's one that I've found myself using it for which utilizes substring()
and toUpperCase()
.
// Index 0123456789 ......
const myString = "the programmer had a lot of bugs in his house";
// Capitalize the first letter of the sentence
const substr =
myString[0].toUpperCase() + myString.substring(1, myString.length);
console.log(substr); // The programmer had a lot of bugs in his house
myString[0]
retrieves the first letter of the string. We then take that value and make it uppercase using toUpperCase()
. We then use the substring()
method to get the remainder of the string (start at index 1, which is the second letter and end at the final letter, which should have an index equal to the length of the string). Finally, we "add" or "concatenate" these two strings together.
trim
This method will "trim" the whitespace off the ends of a string. It may not seem apparent why this is useful, but sometimes, when you grab data from an external API or database, you can't be sure whether the format of that data will be correct.
For example, let's say you get the following data from an external API.
const externalData = [
{
title: "How to code ",
author: " Zach",
},
{
title: " What is Vim?",
author: " Zach",
},
{
title: " How do loops work in JavaScript? ",
author: " Zach",
},
];
The data itself looks fine, but you've got some extra spaces that don't need to be there. Here's how you fix it using trim()
and a basic for loop (see last lesson).
const externalData = [
{
title: "How to code ",
author: " Zach",
},
{
title: " What is Vim?",
author: " Zach",
},
{
title: " How do loops work in JavaScript? ",
author: " Zach",
},
];
for (let i = 0; i < externalData.length; i++) {
const currentTitle = externalData[i].title;
const currentAuthor = externalData[i].author;
externalData[i].title = currentTitle.trim();
externalData[i].author = currentAuthor.trim();
}
console.log(externalData);
If you run this code, you'll see that the objects in the array no longer have spaces around them. It is important to note that trim()
only removes the spaces at the beginning and end of the string; not the spaces between the words. That is why our titles still have those spaces.
match
So... Remember how we talked about those things called "regular expressions" a couple hundred words ago? Well, they're back. Again.
The match()
method is very similar to the exec()
method we talked about with regular expressions. Let's look at them both for comparison.
const regex = /[A-Za-z ]+[0-9]+/;
const str = "my favorite food is steak 239042038124";
// Using the exec() method
const result1 = regex.exec(str);
// Using the match() method
const result2 = str.match(regex);
/*
Both result1 and result2 equal:
["my favorite food is steak 239042038124", index: 0, input: "my favorite food is steak 239042038124", groups: undefined]
*/
These two methods will return the same exact value as long as you are NOT using the global flag in your regular expression.