What are JavaScript Array Methods?
And we are on to arrays! The same rules apply for this section–I'm not intending to provide an exhaustive list with exhaustive explanations; just giving an overview of some of the most common methods.
Here are the array methods that I find myself using most. Please note that most of them require a decent understanding of callback functions, so be sure to re-read the section above if you're still fuzzy on those.
- push() / pop() / shift() / unshift()
- slice()
- splice()
- findIndex() / indexOf()
- map()
- forEach()
- includes()
- filter()
- reduce()
Remember, these are not to memorize!. You can always look them up. I'm showing them to you so that you are aware of them and can identify when they might be useful.
My goal here is not to detail every last thing you can do with each method. The documentation already does that. My goal is to point out why you might want to use these methods.
push, pop, shift, unshift
Official docs - push, pop, shift, unshift
These are related. They let you add/remove elements from an array. From my experience, push()
is the most common method that you will use.
const arr = [1, 2, 3];
// Add element to end of array
arr.push(4); // New array: [1, 2, 3, 4]
// Add element to beginning of array
arr.unshift(0); // New array: [0, 1, 2, 3, 4];
// Remove last element of array
arr.pop(); // New array: [0, 1, 2, 3]
// Remove first element of array
arr.shift(); // New array: [1, 2, 3]
slice
The arr.slice()
method comes in handy when you need to make a copy of an array. To a beginner this may seem useless, but when you start dealing with immutable state in a front-end framework like React, this method will be invaluable to you.
const myArr = ["please", "subscribe", "to", "my", "YouTube channel"];
const fullCopy = myArr.slice(); // ['please', 'subscribe', 'to', 'my', 'YouTube channel']
const partialCopy = myArr.slice(0, 1); // ['please', 'subscribe']
splice (not to be confused with slice)
If you want to add an element somewhere other than the beginning (unshift()
) or end (push()
) of an array, splice()
is your method. Here is a common way to use it. See the docs for more use cases.
// Index 0 1 2 3 4 5
const somethingDoesntBelong = [1, 2, 3, "oops", 5, 6];
// Start at index 3, delete 1 item, and replace with the number 4
somethingDoesntBelong.splice(3, 1, 4);
console.log(somethingDoesntBelong); // [1, 2, 3, 4, 5, 6]
findIndex / indexOf
The findIndex
method accepts a callback function as an argument and will find the first element in an array that matches the conditions set in your callback function.
The indexOf
method simply searches for the first occurrence of a value in an array and is a much simpler method to use.
Let's start easy with the indexOf
method. This just locates a value in an array, and if it doesn't find it, returns -1
.
const arr = ["red", "blue", "green"];
const blueIndex = arr.indexOf("blue"); // 1
const purpleIndex = arr.indexOf("purple"); // -1
But what if you have a more complex array like this?
const moreComplexArr = [
{
firstName: "Bob",
lastName: "Smith",
},
{
firstName: "Alice",
lastName: "Smith",
},
{
firstName: "Jon",
lastName: "Smith",
},
{
firstName: "Jon",
lastName: "Doe",
},
];
How do we find the person with a last name of "Doe"? You might think about trying something like this:
// Using array from above
const valueToFind = {
firstName: "Jon",
lastName: "Doe",
};
// DOESNT WORK!!! Returns -1
const resultIndex = moreComplexArr.indexOf(valueToFind);
This doesn't work because checking the equality of an object is a bit more complex than just passing it in as a value.
With findIndex
, we can locate this element.
const moreComplexArr = [
{
firstName: "Bob",
lastName: "Smith",
},
{
firstName: "Alice",
lastName: "Smith",
},
{
firstName: "Jon",
lastName: "Smith",
},
{
firstName: "Jon",
lastName: "Doe",
},
];
const incorrectIndex = moreComplexArr.indexOf({
firstName: "Jon",
lastName: "Doe",
});
// THIS DOES WORK
const correctIndex = moreComplexArr.findIndex((arrItem) => {
return arrItem.lastName === "Doe";
});
console.log(incorrectIndex); // -1
console.log(correctIndex); // 3
The findIndex()
method provides a lot more flexibility!
map
Of all these built-in array methods, this one is probably my most used. Here is a very practical example. Let's say you have retrieved an array of blog posts from your database and the category
property is not filled out. For all these blog posts, you want them to be categorized in the "Learn to Code" category.
const blogPostsFromDatabase = [
{
title: "How to use the map() function",
category: "uncategorized",
},
{
title: "What is JavaScript?",
category: "uncategorized",
},
{
title: "Why are you crazy enough to learn to code?",
category: "uncategorized",
},
];
function ourCustomCallback(blogPost) {
blogPost.category = "Learn to Code";
return blogPost;
}
const resultingArray = blogPostsFromDatabase.map(ourCustomCallback);
/*
Here is our resultingArray
[
{
title: 'How to use the map() function',
category: 'Learn to Code'
},
{
title: 'What is JavaScript?',
category: 'Learn to Code'
},
{
title: 'Why are you crazy enough to learn to code?',
category: 'Learn to Code'
},
];
*/
The map method can be used in a TON of different situations. If you ever have an array where each element of the array needs to be modified in a similar way, the map method will come in handy.
forEach
So far, I have only shown you how to write a basic for loop. Here is what we have looked at:
const arr = [1, 2, 3];
for (let i = arr.length; i++) {
// Do something with each element of array
}
But there is a simpler way to write this same for loop–the forEach()
Array method.
Please read this for a comparison of the basic for
loop and the forEach
loop. The short answer–each way has its advantages, and in most cases, which one you choose does not matter.
Here is the basic way to use this.
const arr = [1, 2, 3];
let sum = 0;
// We aren't using the `indexOfItem`, but I wanted to put it here to show that it is available to you
function myCallbackFunc(arrItem, indexOfItem) {
sum = sum + arrItem;
}
arr.forEach(myCallbackFunc);
console.log(sum); // 6
Here is a cleaner (but less beginner-friendly) way to write this. Here, we are using an arrow function as the callback rather than defining it separately.
const arr = [1, 2, 3];
let sum = 0;
arr.forEach((arrItem) => {
sum += arrItem;
});
console.log(sum); // 6
includes
If you ever need to figure out whether a value exists in an array, use this method. Please note that you cannot use this to find complex data types like objects or other arrays.
Let's say that you have the following array, and you want to figure out whether the color orange
exists in it. You can clearly see that it does, but you won't always have this clarity while writing code. Maybe this array came from a database and you don't know what to expect!
const myColors = ["blue", "red", "purple", "orange", "green"];
Here is one way that we could figure it out:
const myColors = ["blue", "red", "purple", "orange", "green"];
let orangeExists = false;
for (let i = 0; i < myColors.length; i++) {
if (myColors[i] === "orange") {
orangeExists = true;
}
}
console.log(orangeExists); // true
And here is a simpler way to do it.
const myColors = ["blue", "red", "purple", "orange", "green"];
let orangeExists = false;
myColors.forEach((color) => {
if (color === "orange") {
orangeExists = true;
}
});
console.log(orangeExists); // true
But by using includes()
, we can do it even simpler:
const myColors = ["blue", "red", "purple", "orange", "green"];
let orangeExists = myColors.includes("orange");
console.log(orangeExists); // true
Furthermore, you could have even used a different method altogether to achieve this. See below:
const myColors = ["blue", "red", "purple", "orange", "green"];
let orangeExists = myColors.indexOf("orange") !== -1;
console.log(orangeExists); // true
We know that if indexOf
does NOT find the element in the array, it returns a value of -1
. I know this because I read the documentation.
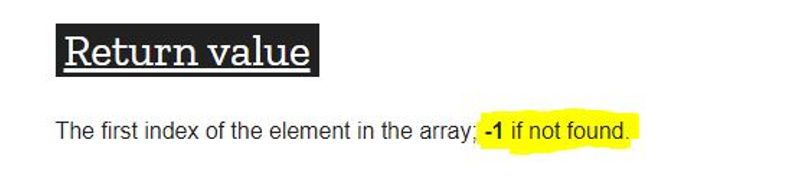
We can use this logic to determine if a value exists in an array; similar to the includes()
method.
Hopefully, you are starting to see how much code these built-in methods can save you from writing if you know when to use them!
filter
Behind map()
, this might be my second most utilized built-in array method.
Unlike some of these other methods, the filter()
method has a very obvious use-case that most people can resonate with even if they don't write a lot of code.
Let's say we are building the "My Orders" page for Amazon. On this page, you can view all of your past orders, but you can also filter by various conditions. You can display orders for a certain time frame, your open orders, your digital only orders, and your canceled orders.
When Amazon loads the data into this page, it likely comes in the form of an array (this is a fictional representation):
const allOrders = [
{
productName: "Tea pot",
isDigital: false,
isCancelled: false,
isOpen: false,
},
{
productName: "Blue Gildan Mens Hoodie",
isDigital: false,
isCancelled: true,
isOpen: false,
},
{
productName: "Code Complete Kindle Book",
isDigital: true,
isCancelled: true,
isOpen: false,
},
{
productName: "Atomic Habits Kindle Book",
isDigital: true,
isCancelled: false,
isOpen: false,
},
];
When the user clicks the filter to select only the Digital orders, how might we write the code to do that? Using the filter()
method of course! We can also get an array with combined filters!
Here's how it works–if the return value of our callback function is true for a specific array item, then this array item will be included in the resultant array.
const digitalOrders = allOrders.filter((orderItem) => {
return orderItem.isDigital;
});
const digitalCancelledOrders = allOrders.filter((orderItem) => {
return orderItem.isDigital && orderItem.isCancelled;
});
const physicalOrders = allOrders.filter((orderItem) => {
return !orderItem.isDigital;
});
You will use this method a lot, so learn it well!
reduce
I saved the hardest for last because while it can come in handy, you don't need it. Take a look at the example, but don't stress over learning this–we have more important things to learn over the next few lessons.
You probably recognize this by now:
const arr = [10, 20, 30, 25, 14];
let sum = 0;
for (let i = 0; i < arr.length; i++) {
sum += arr[i];
}
console.log(sum); // 99
The reduce()
method is just a shorter way of writing this code.
Here is the same code from above re-written using the reduce()
method.
const arr = [10, 20, 30, 25, 14];
function reducerCallback(sum, currArrItem, currArrIndex) {
return (sum += currArrItem);
}
// 0 represents the "initial value"
const result = arr.reduce(reducerCallback, 0);
console.log(result); // 99
We start our sum
value at 0
by passing it in as the second argument (just like we did in the code prior to this). The reducerCallback
will loop through each value in the array and increment the value of sum
by each item in the array. This callback function will then return the "accumulated" sum
.
But what if a method doesn't exist for what I'm trying to do?
Glad you asked. In some cases, you might want to perform some operation that cannot be done using the built-in JavaScript methods.
In that case, you have two options:
- Write a bunch of JavaScript code to solve the problem
- Use a JavaScript "library"
If you tried to compare the equality of objects by writing "Vanilla" (plain) JavaScript code, here's what you would need to write. I don't recommend it.
The better solution is to use a code library like Lodash. We will talk a lot more about code libraries and how to use them later, but for now, just take a glance at the code I've written below (utilizing the Lodash library).
Quick tip: The Lodash library provides functions for various data types (similar to the JavaScript built-in functions) and we often refer to it as "functional programming".
// Don't worry, we have not covered this yet and I don't expect you to know it
const lodashLib = require("lodash");
// As a side-note, the "convention" that a lot of programmers use to import
// this library is to use an underscore as the name of it. You will see this a lot.
// const _ = require('lodash');
const objA = {
prop1: "value",
prop2: 20,
};
const objB = {
prop1: "value",
prop2: 20,
};
console.log(objA === objB); // false (you can't compare equality of JS objects)
// If we tried to implement this ourselves, it would take 100s of lines of code
lodashLib.isEqual(objA, objB); // true
In future lessons, we will walk through how to use a library like this.