Welcome to one of the most important concepts in all of web development.
The distinction between "client" and "server" may seem simple on the surface, but once you start working in the field of web development, you'll realize that it's like an onion—it has many layers (literally—check out the OSI Model).
Why is this concept important to know?
The distinction between client and server is about "context" and "environments".
Think of it like summer vs. winter seasons.
In summer and winter, there are certain things you do and don't do.
In the summer, you have to make sure and put sunblock on, drink lots of water to stay hydrated, and wear light clothing.
In the winter, you have to dress warmly and watch out for ice on the roads!
Similar to this analogy, when we're writing code "client-side" vs. "server-side", there are different things we need to watch out for.
For example, when programming client-side, we must avoid exposing API secret keys! When programming server-side, we have to think more about the performance of our code. Since we're in different "contexts" or "environments", we have different things to worry about!
What is the client-server model?
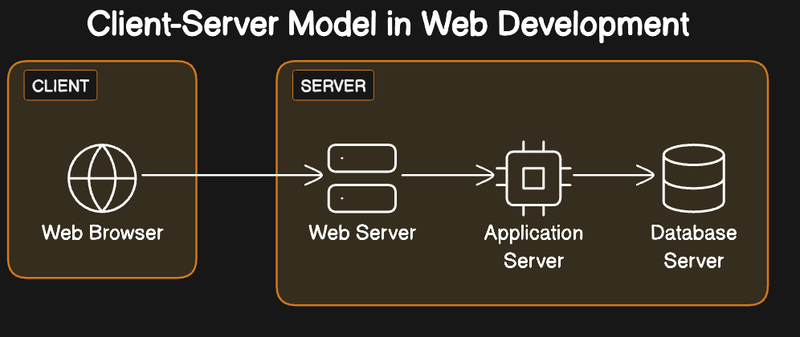
From a formal standpoint, the client-server model is a generic paradigm we use in computing to describe a situation where two parties must communicate in a "requestor" and "responder" setting.
In this post, we'll be looking at this model from the perspective of web development.
Who is the "Client" and "Server"?
In web development, the client can be many things. A client could be...
- A web browser (most common)
- A command-line tool (e.g. curl utility)
- IoT device
- Mobile or desktop application
The key factor here is the role the client plays.
The client is who makes the request for resources.
The server is who responds with those resources.
In other words, the client talks and the server listens.
A server generally comes in the following forms:
- Web servers
- Database servers
- Email servers
In summary, when trying to identify client and server, always ask yourself—who is making the request and who is responding to that request?
The difference between "client-side" and "server-side"
When distinguishing between "client" and "server", we ask ourselves what role the device plays.
When distinguishing between "client-side" and "server-side", we have to ask where the code is running.
In its simplest form, when we are working "client-side", the code is running on the client. When working "server-side", the code is running on the server.
We often refer to these locations as different "contexts" or different "runtimes".
A great example of this is the JavaScript language, which is capable of executing both client-side and server-side.
When JavaScript runs on the client, it is executing in the "browser runtime" (i.e. Google Chrome, Safari, etc.). When JavaScript runs on the server, it is executing in the "Node.js runtime" (a popular server-side JavaScript runtime software).
How do the client and server communicate?
Depending on who the client and server are, they will have a different way to communicate.
Here are just a few examples:
- Hypertext Transfer Protocol (HTTP)—the most common form of communication on the internet where the browser makes an "HTTP request" (typing a domain into the address bar) and the server responds with an "HTTP Response" (the webpage)
- File Transfer Protocol (FTP)—often used with web frameworks like WordPress where a site owner can use an "FTP Client" such as FileZilla to connect to and update website files on their web server
- Simple Mail Transfer Protocol (SMTP)—if you have an iPhone, you may have used Apple Mail. This is the "client" that connects to various email servers (such as Gmail)
As you can see, in all cases, the method of communication between the client and server must be standardized. Similar to how most people in the country of France speak French as a shared way to communicate, computers use protocols like HTTP, FTP, and SMTP to communicate in different contexts with each other.
Client Side Development Deep Dive
Let's take a deeper look at what makes "client-side" web development special.
What languages are used client-side?
As a rule of thumb, when someone says "client-side", they are generally talking about a client that runs the following 3 languages:
- HTML
- CSS
- JavaScript
That said, remember, the "client" can be many different things. If the client is a web browser, it will use HTML, CSS, and JavaScript to show a webpage.
But if our "client" is a command-line tool, it may use many other languages!
For example, if you are using the Bash shell, that is written in the C language.
Who writes client-side code and what are they responsible for?
In general, a more common term for a developer that primarily writes client-side code is a "frontend web developer".
A frontend developer is a type of web developer who specializes in designing and building the user interface (UI) and user experience (UX) of a website or web application.
When we talk about client-side development, there are many sub-disciplines involved which include things like:
- Rendering HTML and CSS
- User interactions
- Forms
- Loading states
- Navigation and routing
What are the advantages and limitations of client-side code?
For the sake of simplicity, we'll talk about web browsers and ignore the fact that a client can be other things.
When talking about web browsers like Google Chrome, there are several advantages we have:
- Lots of interactivity—we can prompt and respond to user events directly, which is a fast and interactive experience for the end user
- Cheap—in most cases, the code a frontend developer writes will execute on somebody else's computer (browser)! This is a double-edged sword. On one hand, if the frontend developer writes slow and bad code, they don't have to pay money for the mistakes they made. That said, the user experience will be bad and they will pay by losing their users!
On the flip side, there are some disadvantages to client-side development:
- Security concerns—since your code is running on someone else's web browser, that means you can't publish secret or confidential information client-side. Security is a top priority to avoid hacks.
- Browser compatibility—every user has a preference for what browser they use. Some users prefer Google Chrome while others prefer Safari. Since each browser has slight differences in its implementations, it can often be a burden to write client-side code and keep it working on all types of user devices!
- You are dependent on your user's device—if your user has bad Wifi or an old phone that doesn't work well, your app may not run as you originally intended! As a result, when developing client-side applications, it is important to keep your "bundle size" low (i.e. how much JavaScript code is required for the app to run)
Example client-side code
// JavaScript to display an alert box with a message
document.getElementById("myButton").addEventListener("click", function() {
alert("Hello, world!");
});
This snippet adds an event listener to a button with the ID myButton
. When the button is clicked, it displays an alert box saying "Hello, world!". This code runs entirely in the browser, and it directly manipulates the webpage's DOM (Document Object Model).
Server Side Development Deep Dive
Time to take a look at what server-side development is all about!
What languages are used server-side?
Unlike client-side development where there are a small set of languages used, server-side development comes with a huge list of languages.
Some of the most popular languages that "can run on a server" are:
- JavaScript (Node.js)
- Python
- Java
- PHP
- Ruby
- C#
- Go
- TypeScript
- Swift
- Kotlin
- Perl
- Scala
- Rust
- Elixir
- F#
- Dart
- Haskell
- Clojure
- Lua
- Erlang
And that's just a handful of the hundreds of programming languages out there!
Each has its strengths and weaknesses. For example, JavaScript is great if you're building full-stack web applications while Elixir is an excellent server-side language for embedded systems.
Who writes server-side code and what are they responsible for?
The more common term for a developer that writes server-side code is a "backend developer".
Backend developers typically work on the business logic of applications and would include tasks like:
- Data processing and storage
- Client request handling
- Database interaction
- Application logic implementation
- API integration
- Security measures implementation
- Session management
- Data validation and sanitation
- Error handling and logging
- File management and manipulation
Example server-side code
// Node.js to create a simple web server that responds with 'Hello, World!'
const http = require('http');
http.createServer(function (req, res) {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello, World!\n');
}).listen(3000);
console.log('Server running at http://localhost:3000/');
This Node.js script creates a web server that listens on port 3000. When a request is made to this server, it responds with "Hello, World!" in plain text. This code runs on the server and unlike client-side code, doesn't have access to the client's DOM. It's responsible for handling HTTP requests and sending responses to the client.
The key thing to remember here is that this code is executed on the Node.js "runtime". Remember, client-side vs server-side is about where the code executes!
Key differences between client-side and server-side
Having seen each in isolation, let's bring it full circle and talk about the key differences between client-side and server-side programming.
Distinction #1: Where the code executes
Client-side code runs in the user's browser, within the browser's runtime environment. JavaScript is a common example, used for creating interactive web pages. Imagine clicking a button to display a form - this is JavaScript executing within the browser's runtime.
Server-side code, on the other hand, executes on the server within a server runtime environment, like Node.js for JavaScript or a Python interpreter for Python code. An example is the login process. When you submit credentials, the server-side code, perhaps written in Python, processes this request within its runtime, securely interacting with the server's resources.
Distinction #2: Security considerations
Client-side code is more vulnerable to security risks as it's exposed to the user. This means anyone can view, modify, or manipulate the code using tools like browser developer tools. For example, JavaScript code for form validation can be seen and altered, potentially leading to unauthorized actions or data exposure.
Server-side code, in contrast, offers enhanced security. It runs on the server and is not directly accessible to the end user. This isolation from the client's environment means that critical operations, like database transactions, user authentication, and data processing, are less susceptible to tampering or exposure.
For instance, when processing a payment, the server-side code securely handles sensitive data like credit card information, ensuring that it's encrypted and safely transmitted, far from the reach of client-side vulnerabilities. This separation not only protects sensitive data but also maintains the integrity of the application's core logic, safeguarding against various web security threats like Cross-Site Scripting (XSS) and SQL Injection.
Distinction #3: Purpose of the code
Client-side code typically handles user interaction, layout, and presentation. For example, using CSS and JavaScript for dynamically changing the look of a webpage in response to user actions.
Server-side code, however, deals with data management, business logic, and server configuration. It could involve querying a database and returning data, like fetching user profiles or processing e-commerce transactions.
An Example of a Client/Server Interaction

To help all these concepts stick, let's look at a specific, real-world example of the "client/server boundary".
In the flow below, imagine that you are sitting at your computer using the Google Chrome web browser to load YouTube and watch a video. Here's what happens (pay attention to client-side vs server-side labels):
- Client-Side Action: URL Request
- You type 'youtube.com' into the Google Chrome address bar and hit enter. This is a client-side action where your browser prepares to request information from YouTube's servers.
- Server-Side Action: Processing the Request
- YouTube's server receives your request. This is a server-side action where the server interprets your request and prepares the appropriate response. Server-side code, perhaps in Python, is responsible for this processing.
- Server-Side Action: Sending Data
- The server responds by sending the HTML, CSS, and JavaScript files back to your browser. This is a server-side action, involving the transmission of data from the server to your client.
- Client-Side Action: Rendering the Webpage
- Your browser (Google Chrome) receives these files and renders the YouTube homepage. This involves using HTML and CSS for layout and design, and JavaScript for interactivity. All these are client-side actions, as they happen in your browser.
- Client-Side Action: Requesting Video Data
- When you click on a video, JavaScript in your browser handles this action and sends a request back to the server for that specific video's data. This is a client-side action.
- Server-Side Action: Retrieving and Sending Video
- The server receives the request for the video and processes it. The server-side code fetches the video file and related data, like comments, and sends it to the client. This is a server-side action.
- Client-Side Action: Streaming and Displaying the Video
- Your browser receives the video data and starts streaming the video. The controls for the video (play, pause, volume) are also managed by client-side JavaScript.
- Client and Server-Side Interaction: User Interactions
- As you interact with the website (like liking a video or commenting), these actions are initiated client-side by JavaScript. The browser sends these actions to the server. The server processes these actions (like updating the like count or storing your comment) and sends back any needed responses. This involves both client-side and server-side actions in a continuous interaction loop.
Summary
In summary, here's a short list of ideas to help you remember the difference between client side and server side.
- Client-side is "frontend" and server-side is "backend"
- Client-side vs. server-side is about who (made the request/response) and where (the code executes)
- The client makes the request. The server responds.
- The client talks; the server listens.
- A "client" can be many things. Most often we are referring to a web browser, but it could also be a desktop app, command line tool, or IoT device.
- A "server" can also be many things. Typically, we're referring to "web servers" (how the internet works), but it could also be a database server, email server, or many other types of servers!